Motor
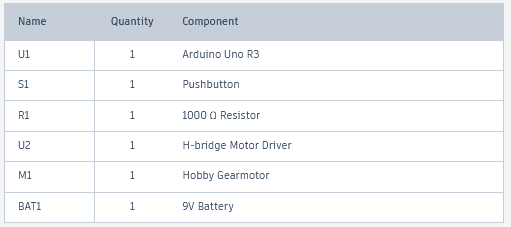
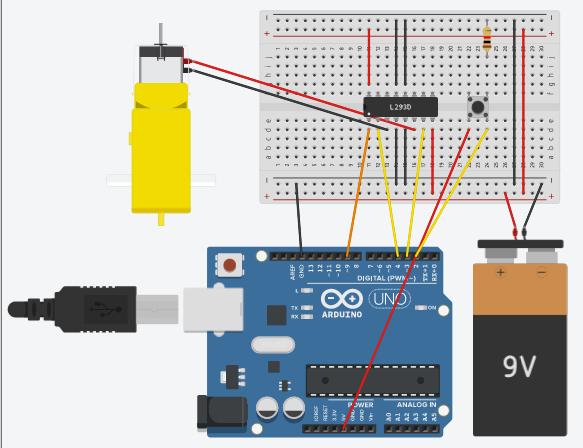
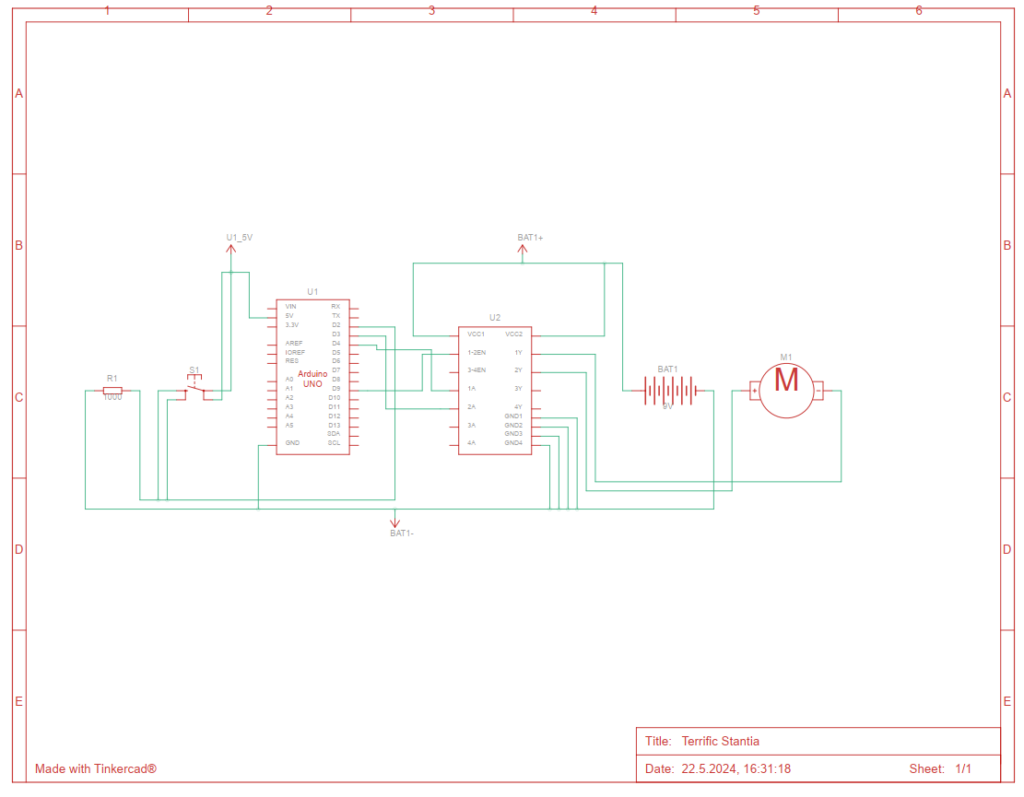
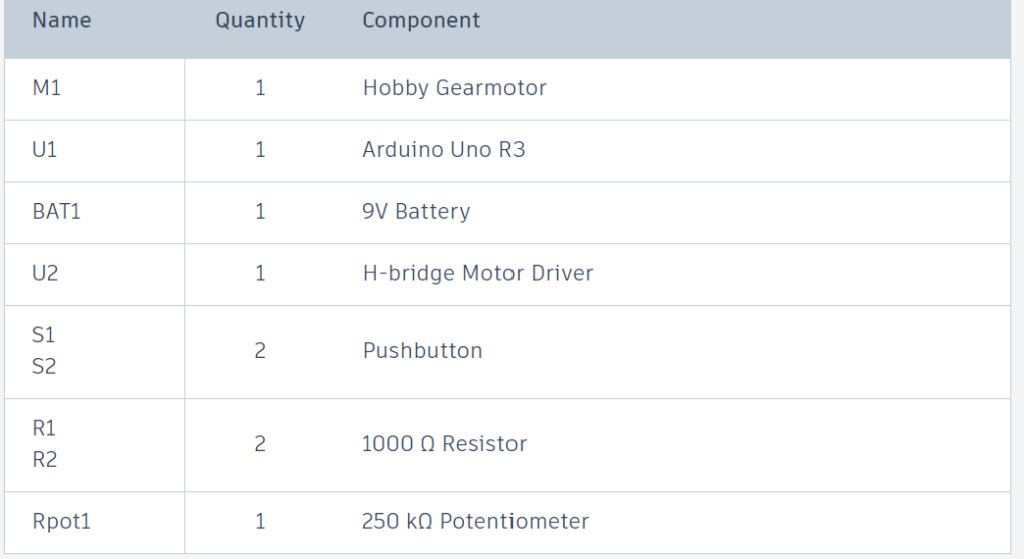

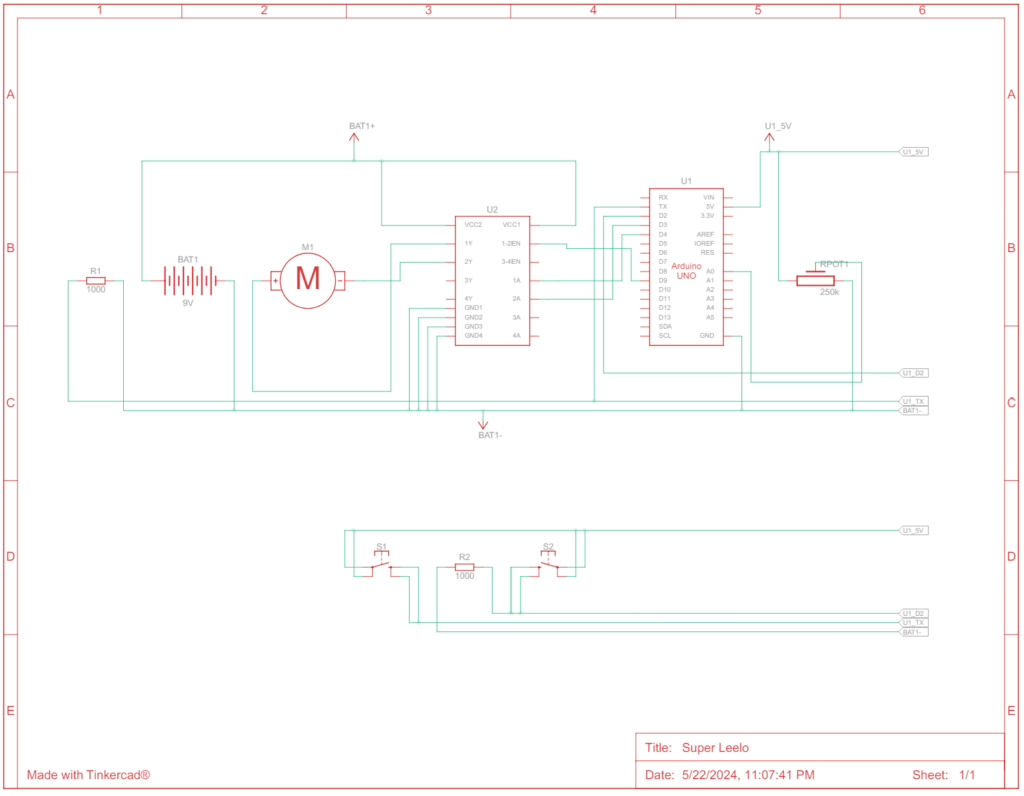
int switchPin = 2; // switch 1
int motor1Pin1 = 3; // pin 2 (L293D)
int motor1Pin2 = 4; // pin 7 (L293D)
int enablePin = 9; // pin 1 (L293D)
void setup() {
// inputs
pinMode(switchPin, INPUT);
// outputs
pinMode(motor1Pin1, OUTPUT);
pinMode(motor1Pin2, OUTPUT);
pinMode(enablePin, OUTPUT);
// enable motor1
digitalWrite(enablePin, HIGH);
}
void loop() {
// if the switch is HIGH, move the motor in one direction:
if (digitalRead(switchPin) == HIGH) {
digitalWrite(motor1Pin1, LOW); // pin 2 (L293D) LOW
digitalWrite(motor1Pin2, HIGH); // pin 7 (L293D) HIGH
}
// if the switch is LOW, move the motor in the other direction:
else {
digitalWrite(motor1Pin1, HIGH); // pin 2 (L293D) HIGH
digitalWrite(motor1Pin2, LOW); // pin 7 (L293D) LOW
}
}
int switchPin = 2; // switch 1
int switchPin2 = 1; // switch 2
int potPin = A0; // potentiometer
int motor1Pin1 = 3; // pin 2 (L293D)
int motor1Pin2 = 4; // pin 7 (L293D)
int enablePin = 9; // pin 1 (L293D)
void setup() {
// inputs
pinMode(switchPin, INPUT);
pinMode(switchPin2, INPUT);
// outputs
pinMode(motor1Pin1, OUTPUT);
pinMode(motor1Pin2, OUTPUT);
pinMode(enablePin, OUTPUT);
}
void loop() {
// motor speed
int motorSpeed = analogRead(potPin);
// activate motor
if (digitalRead(switchPin2) == HIGH) {
analogWrite(enablePin, motorSpeed);
} else {
analogWrite(enablePin, 0);
}
// if the switch is HIGH, move the motor in one direction:
if (digitalRead(switchPin) == HIGH) {
digitalWrite(motor1Pin1, LOW); // pin 2 (L293D) LOW
digitalWrite(motor1Pin2, HIGH); // pin 7 (L293D) HIGH
}
// if the switch is LOW, move the motor in the other direction:
else {
digitalWrite(motor1Pin1, HIGH);
digitalWrite(motor1Pin2, LOW);
}
}
- Pin Definitions:
switchPin
: the pin for the switch.motor1Pin1
: the first pin for controlling the motor (connected to pin 2 on the L293D chip).motor1Pin2
: the second pin for controlling the motor (connected to pin 7 on the L293D chip).enablePin
: the pin for enabling the motor (connected to pin 1 on the L293D chip).
- Setup Function:
pinMode(switchPin, INPUT)
: Sets the switch pin as an input.pinMode(motor1Pin1, OUTPUT)
: Sets the first motor control pin as an output.pinMode(motor1Pin2, OUTPUT)
: Sets the second motor control pin as an output.pinMode(enablePin, OUTPUT)
: Sets the enable pin as an output.digitalWrite(enablePin, HIGH)
: enables the motor by setting the enable pin HIGH.
- Loop Function:
if (digitalRead(switchPin) == HIGH)
: Checks if the switch is pressed (HIGH).else
: If the switch is not pressed (LOW),- Sets
motor1Pin1
to HIGH andmotor1Pin2
to LOW, moving the motor in the opposite direction.
- Sets
———->
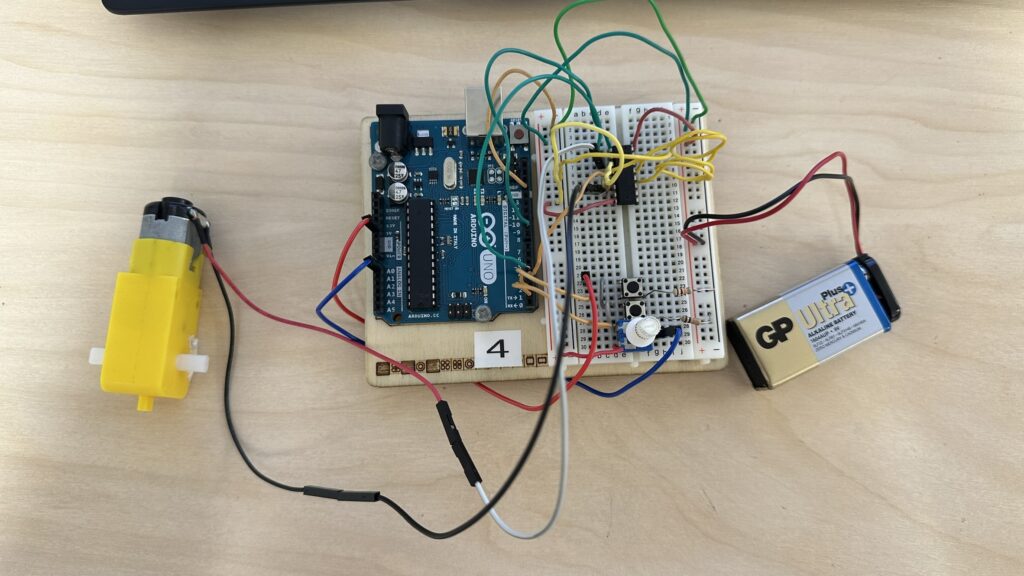
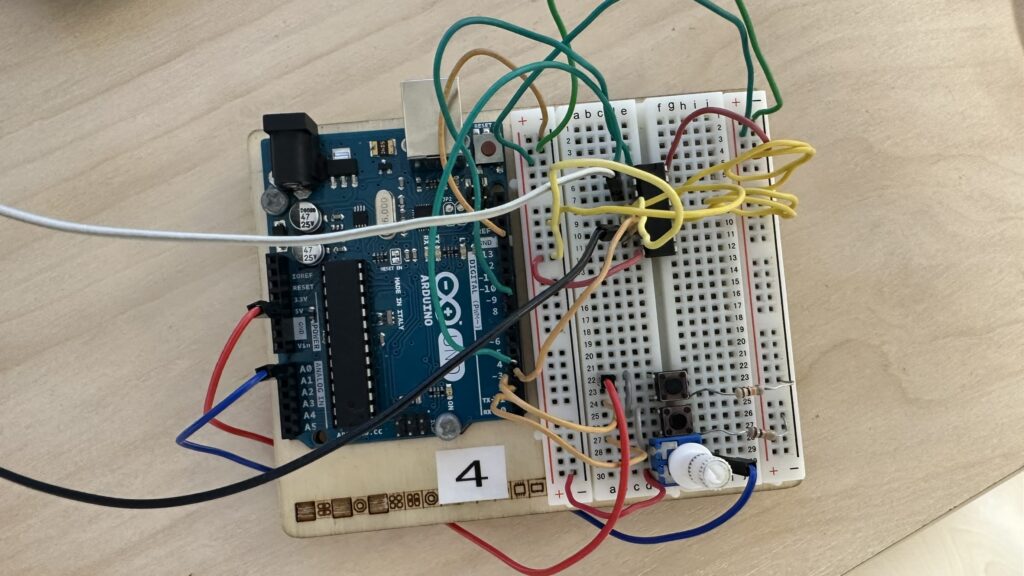
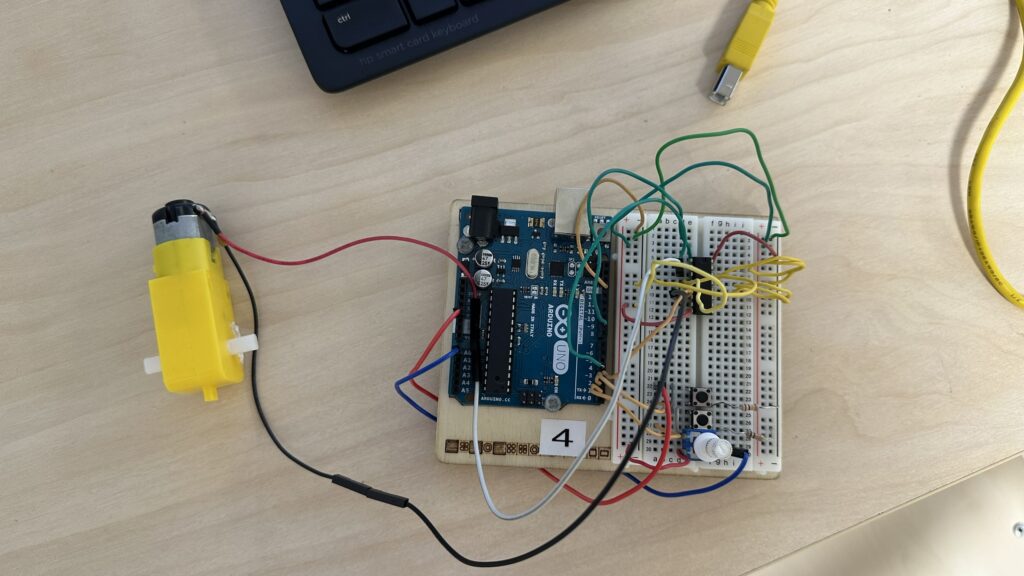
- Pin Definitions:
switchPin
andswitchPin2
: defines the pin for the first switch and fpr the seconf switch.potPin
: the pin for the potentiometer.motor1Pin1
,motor1Pin2
,enablePin
- Setup Function:
pinMode(switchPin, INPUT)
andpinMode(switchPin2, INPUT)
pinMode(motor1Pin1, OUTPUT)
andpinMode(motor1Pin2, OUTPUT)
pinMode(enablePin, OUTPUT)
- Loop Function:
motorSpeed
: Reads the value from the potentiometer to set the motor speed.- Activate Motor:
if (..)
: Checks if the second switch is pressed.analogWrite(enablePin, motorSpeed)
: If pressed, sets the motor speed based on the potentiometer value.
else { analogWrite(enablePin, 0); }
: If not pressed, stops the motor
- Control Motor Direction:
if (..)
: Checks if the first switch is pressed.digitalWrite(motor1Pin1, LOW)
: Sets the first motor control pin LOW.digitalWrite(motor1Pin2, HIGH)
: Sets the second motor control pin HIGH, moving the motor in one direction.
else
: If switch is not pressed,dig
italWrite(motor1Pin1, HIGH)
: Sets the first motor control pin HIGH.digitalWrite(motor1Pin2, LOW)
: Sets the second motor control pin LOW, moving the motor in the opposite direction
Using the distance measurement sensor
#define ECHO_PIN 8
#define TRIG_PIN 7
void setup()
{
pinMode(ECHO_PIN, INPUT);
pinMode(TRIG_PIN, OUTPUT);
Serial.begin(9600);
}
void loop()
{
digitalWrite(TRIG_PIN,HIGH);
digitalWrite(TRIG_PIN,LOW);
int distance=pulseIn(ECHO_PIN, HIGH)/50;
Serial.println(distance);
}
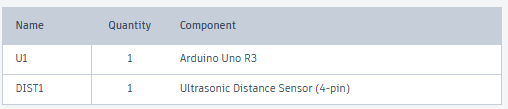
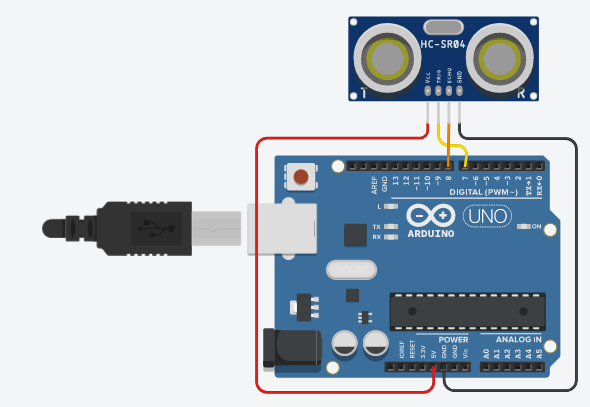
#define ECHO_PIN 8
#define TRIG_PIN 7
void setup() {
pinMode(ECHO_PIN, INPUT);
pinMode(TRIG_PIN, OUTPUT);
Serial.begin(960);
}
void loop() {
Serial.println(measure());
}
int measure()
{
digitalWrite(TRIG_PIN,HIGH);
digitalWrite(TRIG_PIN,LOW);
int distance=pulseIn(ECHO_PIN, HIGH,15000)/50;
return constrain(distance,1,300);
}
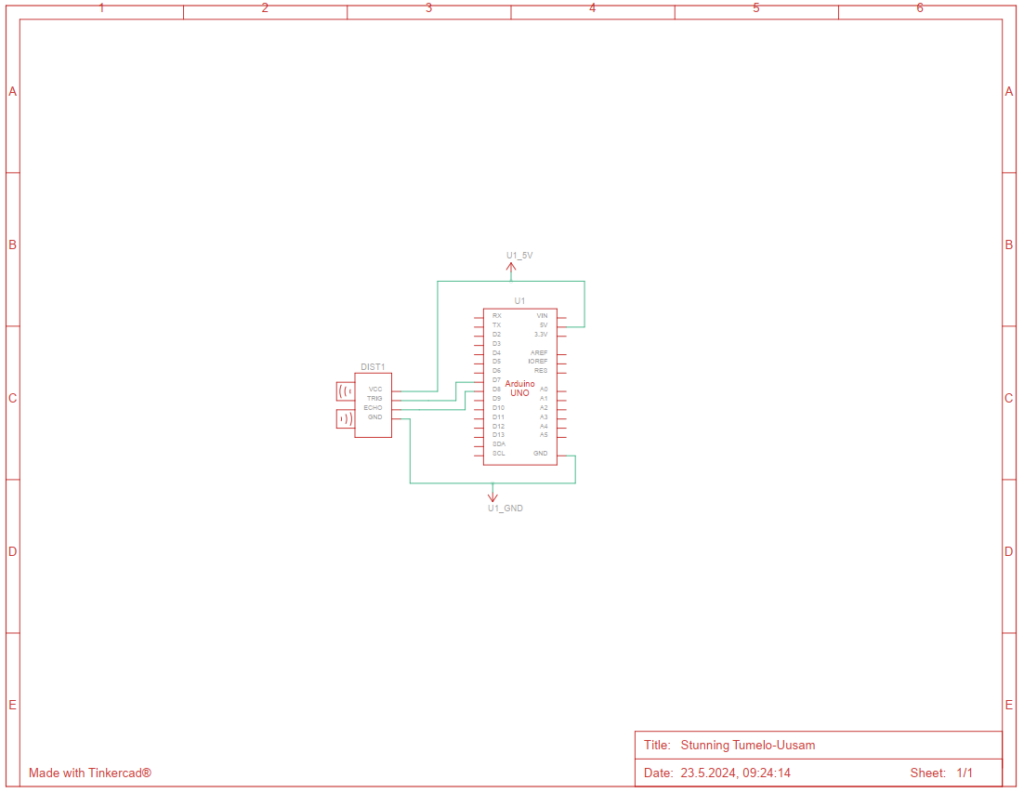
Simple parking system
This code controls a motor, LED, and buzzer based on the distance measured by an distance sensor. If the distance is greater than 50 cm, the motor runs, the LED is off, and the buzzer is silent. If the distance is 50 cm or less, the motor stops, the LED lights up, and the buzzer sounds.

#define ECHO_PIN 7
#define TRIG_PIN 8
int motorPin1=3;
int distance=1;
int LedPin=13;
int duration;
const int buzzerPin = 9;
void setup() {
pinMode(ECHO_PIN, INPUT);
pinMode(TRIG_PIN, OUTPUT);
pinMode(motorPin1,OUTPUT);
pinMode(LedPin,OUTPUT);
pinMode(buzzerPin, OUTPUT);
Serial.begin(9600);
}
void loop() {
digitalWrite(TRIG_PIN,LOW);
delay(200);
digitalWrite(TRIG_PIN,HIGH);
delay(200);
digitalWrite(TRIG_PIN,LOW);
duration = pulseIn(ECHO_PIN, HIGH);
distance=duration/58;
Serial.println(distance);
if (distance>50)
{
analogWrite(motorPin1,100);
digitalWrite(LedPin,0);
noTone(buzzerPin);
delay(1000);}
else
{
analogWrite(motorPin1,0);
digitalWrite(LedPin,250);
tone(buzzerPin, 1000);
}
}
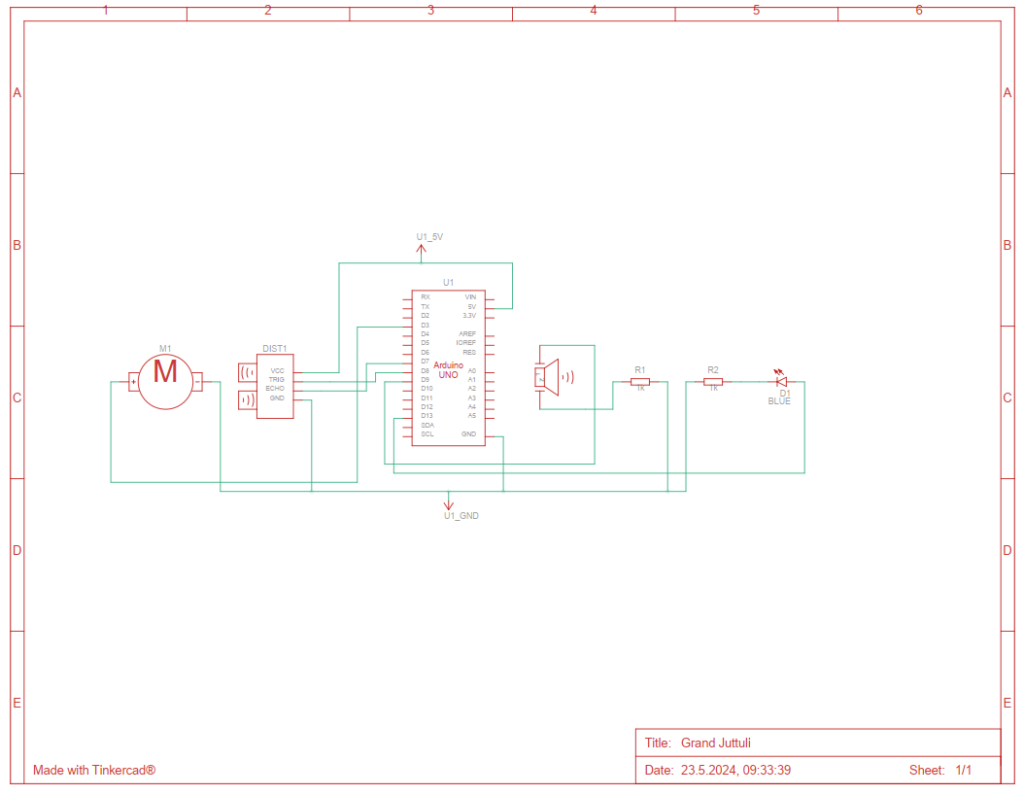
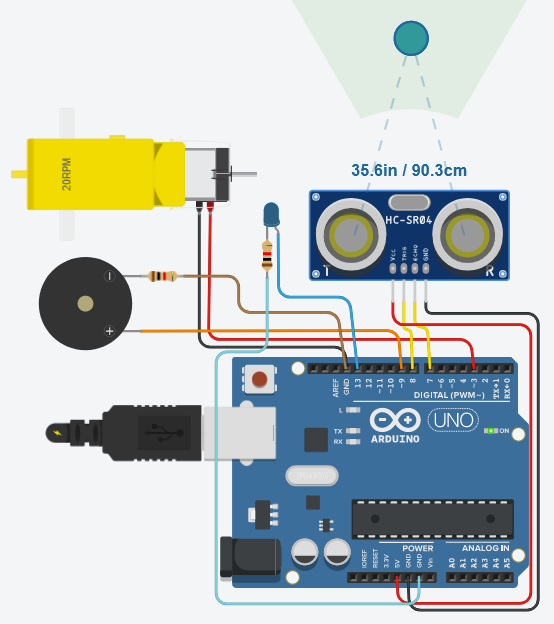
ECHO_PIN
(7) andTRIG_PIN
(8): used for the distance sensormotorPin1
(3): controls the motorLedPin
(13): controls the LEDbuzzerPin
(9): controls the buzzerdistance
(initially 1): stores the measured distanceduration
: stores the time it takes for the distance sensor to return- Setup Function:
- Initializes the pins for input/output
- Begins serial at 9600
- Loop Function:
- Triggers the distance sensor to send a pulse:
- Sets
TRIG_PIN
low, waits 200 ms - Sets
TRIG_PIN
high, waits 200 ms - Sets
TRIG_PIN
low again
- Sets
- Measures the duration returned by the sensor
- Converts the duration to distance in centimeters
- Prints the distance to the Serial Monitor
- Triggers the distance sensor to send a pulse:
- Logic:
- If the distance is greater than 50 cm:
- The motor runs at a speed of 100
- The LED is turned off
- The buzzer is silent
- If the distance is 50 cm or less:
- The motor stops
- The LED is turned on
- The buzzer sounds at a frequency of 1000 Hz
- If the distance is greater than 50 cm:
Trash Can
This code controls a trash can with a servo motor, an distance measurement sensor, an RGB LED, and an LCD display. The distance sensor detects the distance to an object, and if it is close enough, the servo motor opens the trash can lid. The RGB LED changes color based on the state of the trash can (opening, open, closing, closed), and the LCD display shows the number of times the trash can has been opened.
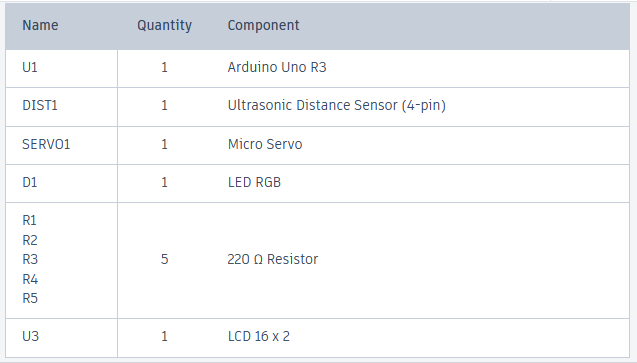
#include <Servo.h>
#include <LiquidCrystal.h>
#define TRIG_PIN 7
#define ECHO_PIN 6
Servo myservo;
const int servoPin = 8;
const int redPin = 13;
const int greenPin = 9;
const int bluePin = 10;
LiquidCrystal lcd(11, 12, 2, 3, 4, 5);
int trashCount = 0;
int distance;
int duration;
void setup() {
pinMode(ECHO_PIN, INPUT);
pinMode(TRIG_PIN, OUTPUT);
myservo.attach(servoPin);
myservo.write(0); // Initial position
pinMode(redPin, OUTPUT);
pinMode(greenPin, OUTPUT);
pinMode(bluePin, OUTPUT);
lcd.begin(16, 2);
lcd.setCursor(1, 0);
lcd.print("Trash Count: ");
lcd.print(trashCount);
delay(1000);
}
void loop() {
digitalWrite(TRIG_PIN, LOW);
delayMicroseconds(2);
digitalWrite(TRIG_PIN, HIGH);
delayMicroseconds(10);
digitalWrite(TRIG_PIN, LOW);
duration = pulseIn(ECHO_PIN, HIGH);
distance = duration / 58.2; // duration to cm
if (distance > 0 && distance < 100) {
lightOn("opening");
openTrashCan();
lightOn("open");
trashCount++;
updateLCD();
delay(5000); // keep the trash opened for 5 seconds
closeTrashCan();
lightOn("closing");
delay(1000); // give some time before marking closed
lightOn("closed");
}
delay(100);
}
void openTrashCan() {
myservo.write(90); // Open position
}
void closeTrashCan() {
myservo.write(0); // Closed position
}
void updateLCD() {
lcd.clear();
lcd.setCursor(1, 0);
lcd.print("Trash Count: ");
lcd.print(trashCount);
}
void lightOn(String state) {
if (state == "closed") {
setLEDColor(255, 0, 0); // Red
} else if (state == "opening" || state == "closing") {
setLEDColor(255, 255, 0); // Yellow
delay(2000);
} else if (state == "open") {
setLEDColor(0, 255, 0); // Green
}
}
void setLEDColor(int red, int green, int blue) {
analogWrite(redPin, red);
analogWrite(greenPin, green);
analogWrite(bluePin, blue);
}
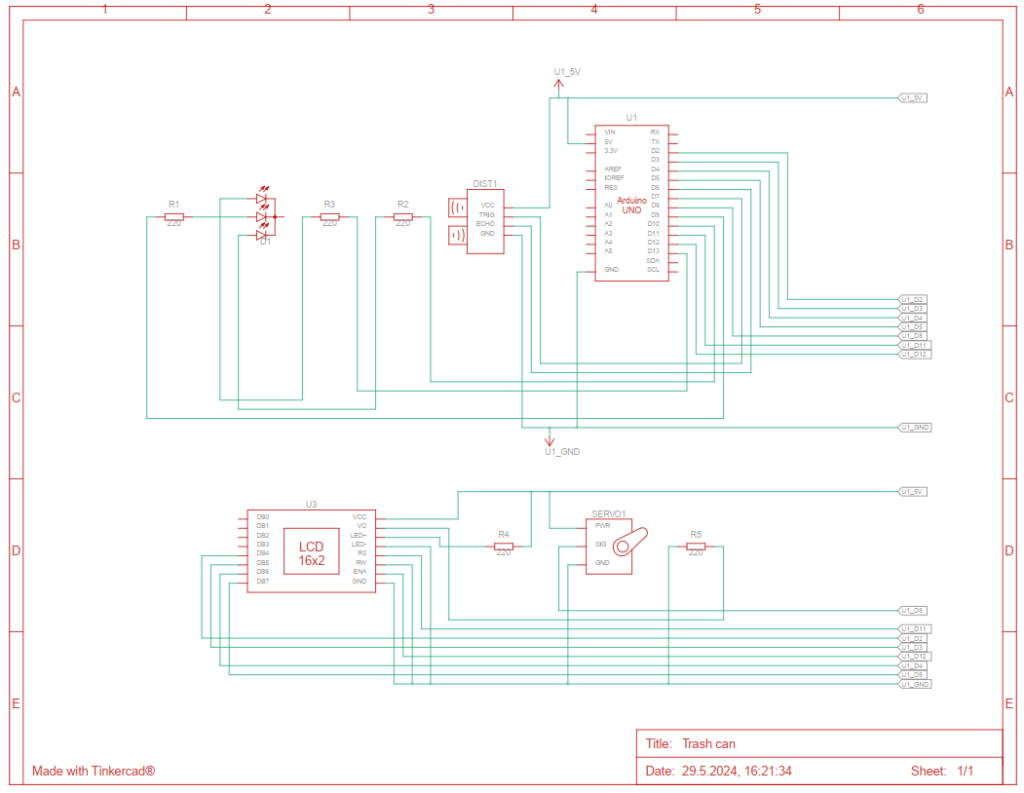
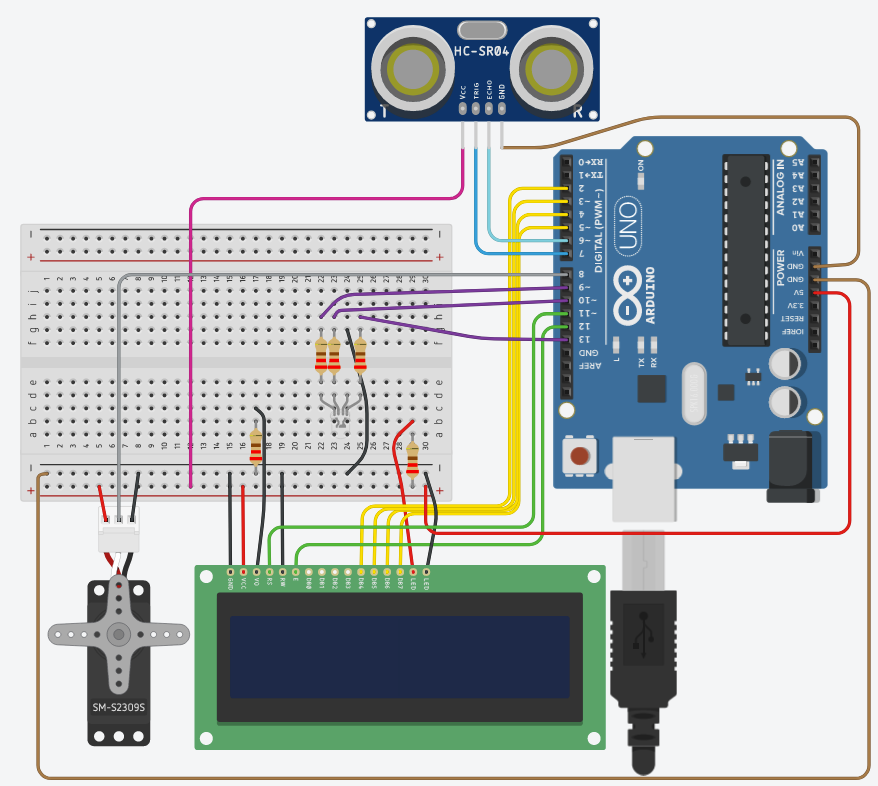
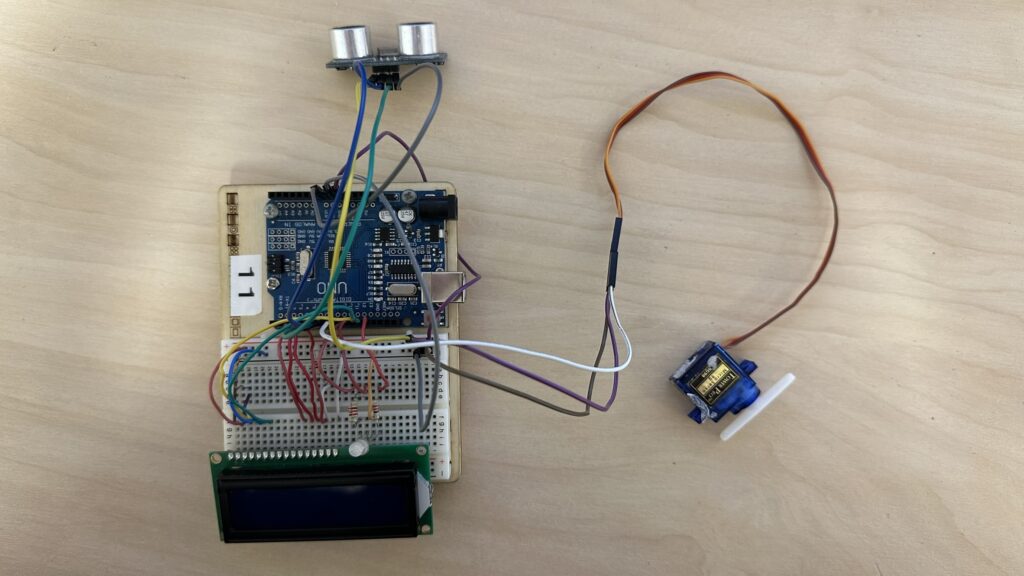
- Include Libraries:
Servo.h
: controls the servo motorLiquidCrystal.h
: controls the LCD display
- Pins:
TRIG_PIN
andECHO_PIN
: used for the distance measurement sensorservoPin
: controls the servo motorredPin
,greenPin
,bluePin
: control the RGB LED
- Objects:
Servo myservo
: creates a servo objectLiquidCrystal lcd
: creates an LCD object with specified pin connections
- Variables:
trashCount
: counts the number of times the trash can is openeddistance
,duration
: used to measure the distance from the distance sensor
- Setup Function:
- initializes pins for input/output.
- connect the servo motor to the
servoPin
and sets its initial position - initializes the RGB LED pins as outputs
- initializes the LCD display and prints the initial trash count, 0
- Loop Function:
- measures the distance using the distance sensor
- if the distance is within 100 cm the trash can opens:
- The LED changes to yellow (“opening”)
- The servo motor moves to open the lid
- The LED changes to green (“open”)
- Increases the trash count and updates the LCD display
- waits for 5 seconds, then closes the lid:
- the LED changes to yellow (“closing”), then red (“closed”)
- Helper Functions:
openTrashCan()
: moves the servo to open the lidcloseTrashCan()
: moves the servo to close the lidupdateLCD()
: updates the LCD with the current trash countlightOn(String state)
: changes the LED color based on the trash can’s statesetLEDColor(int red, int green, int blue)
: sets the RGB LED color