You can find the original instructions here: https://habr.com/ru/articles/529332/
The schedule is being transferred from the Google Sheets to the Google Calendar.
For this, you need:
- Google Account
- Some knowledge of JavaScript! But not entirely sure:)
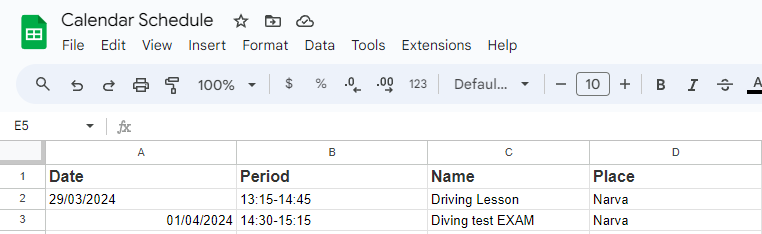
The First step: Create a new table!
You need fields like this:
- Event title
- Event Date
- Event Duration
- Location of the event
The second step: Create a Script”
Go to the Script Editor
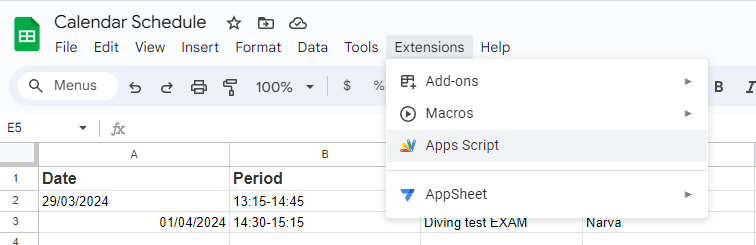
The final step:
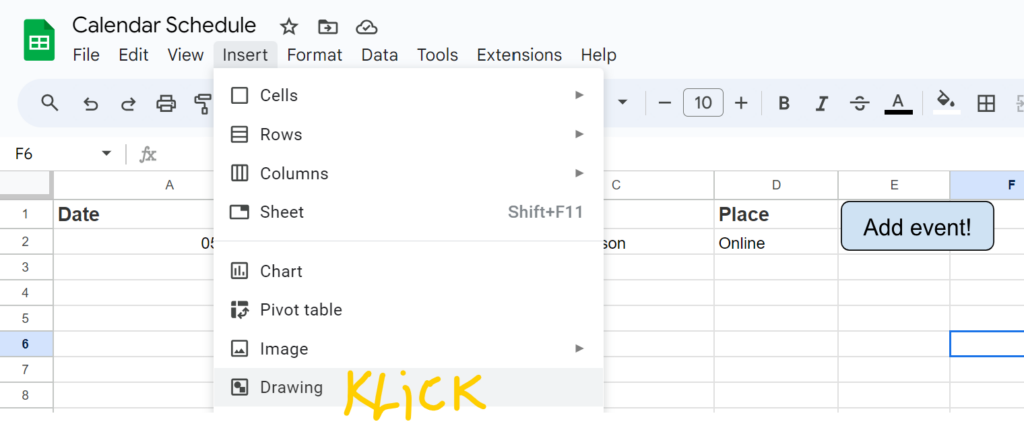

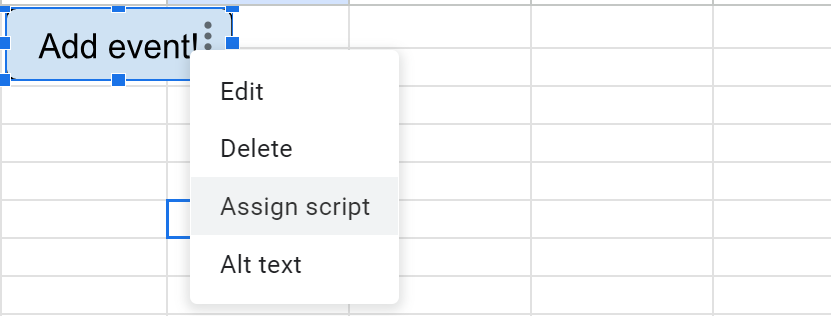
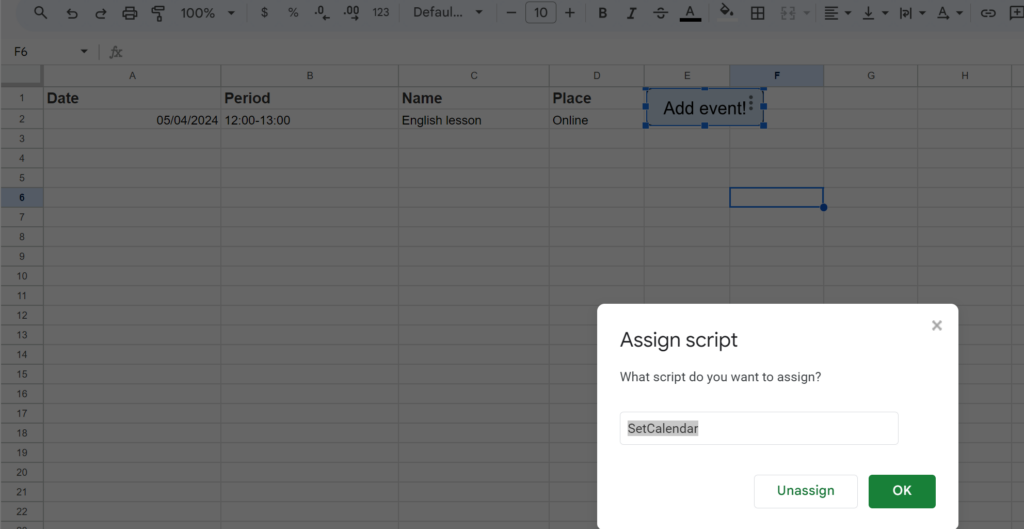
There are different ways to trigger the function, the most convenient one for me seemed to be a button
The code itself:
Extract information about events from the table:
Note! Row and column indexing starts at 1.
function SetCalendar() {
//The indexes of the first row and the first column in the data table
const rowStart = 1;
const colStart = 1;
//The number of rows and columns in the schedule
const colsCount = 4;
const rowsCount = 3;
//Getting the page
var sheet = SpreadsheetApp.getActiveSheet();
//Extracting table data within the specified ranges
var range = sheet.getRange(rowStart, colStart, rowsCount, colsCount)
var data = range.getDisplayValues();
//Constants for column indexes
const dateCol = 0;
const timeCol = 1;
const nameCol = 2;
const placeCol = 3;
//savedDate — In variable, we store the last read date!
let savedDate = "";
//We access the data with variable i, where the loop iterates and retrieves the value
//(which is an index), ultimately returning the row (the current row of the table)
for (var i in data) {
let row = data[i];
let classDate = row[dateCol]; //row[dateCol] — we extract the date from the dateCol column.
let classPeriod = row[timeCol];
let className = row[nameCol];
let classPlace = row[placeCol];
if (classDate.trim() == "") {
//If the date is an empty string, then we take the date from the savedDate variable.
classDate = savedDate;
} else {
//Otherwise, we update the value of savedDate
savedDate = classDate;
}
//We get the start and end date-time of the event
let classTimeInfo = extractPeriod(classPeriod, classDate);
let classStartTime = classTimeInfo.from;
let classEndTime = classTimeInfo.to;
//Merge all additional information
let info = "Place: " + classPlace;
//We access Google Calendar through methods of the global object CalendarApp
//We use the getCalendarsByName method to obtain an array of calendars
//with the specified name, mine is named 'Important Matters'
//From the array, we need to take one element, which is the zeroth [0] element.
var event = (CalendarApp.getCalendarsByName("Important Matters"))[0].createEvent
(
className,
classStartTime,
classEndTime,
{
//description of the event, which we earlier stored in the variable 'info'
description: info
}
);
//As sometimes frequent calls to the Google Calendar service might cause issues,
//it is recommended to introduce a delay between method calls to the calendar
Utilities.sleep(50);
}
}
getDisplayValues()– получаем значения в виде строк
Получаем дату занятия в формате dd/mm/yyyy
function extractTime(timeStr, dateStr) {
//It splits the time string into hours and minutes based on the colon (:) separator.
let sepIdx = timeStr.indexOf(":");
let hoursStr = timeStr.substring(0, sepIdx);
let minsStr = timeStr.substring(sepIdx + 1);
//It splits the date string into day, month, and year based on the slash (/) separator.
sepIdx = dateStr.indexOf("/");
let dayStr = dateStr.substring(0, sepIdx);
let monthStr = dateStr.substring(sepIdx + 1, sepIdx + 3);
sepIdx = dateStr.indexOf("/", sepIdx + 1);
let yearStr = dateStr.substring(sepIdx + 1);
//Note that the month is adjusted by subtracting 1
//since months in JavaScript Date objects are zero-indexed
//(January is 0, February is 1, and so on).
let t = new Date();
t.setHours(parseInt(hoursStr), parseInt(minsStr));
t.setYear(parseInt(yearStr));
t.setMonth(parseInt(monthStr) - 1, parseInt(dayStr));
return t;
}
This function extracts the start and end times of a period from the provided period string and date string using the extractTime
function.
function extractPeriod(periodStr, dateStr) {
//It finds the index of the hyphen '-' in the period string
//to separate the start and end times.
let sepIdx = periodStr.indexOf("-");
let fromStr = periodStr.substring(0, sepIdx);
let toStr = periodStr.substring(sepIdx + 1);
//It trims any leading or trailing whitespace from both time strings.
fromStr = fromStr.trim();
toStr = toStr.trim();
return {
from: extractTime(fromStr, dateStr),
to: extractTime(toStr, dateStr)
}
}
Result!!!
Of course, after launching the function by clicking on the button we created.
If you go to Google Calendar on your computer, it looks like this:
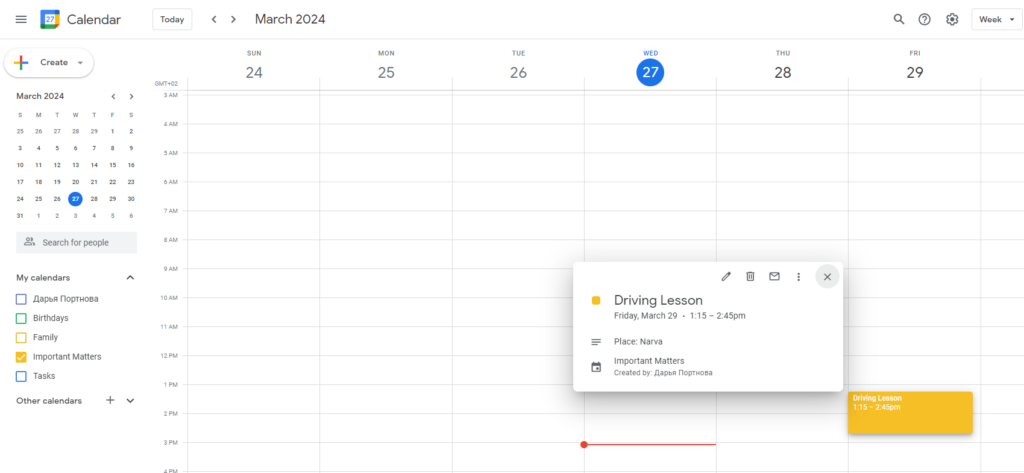
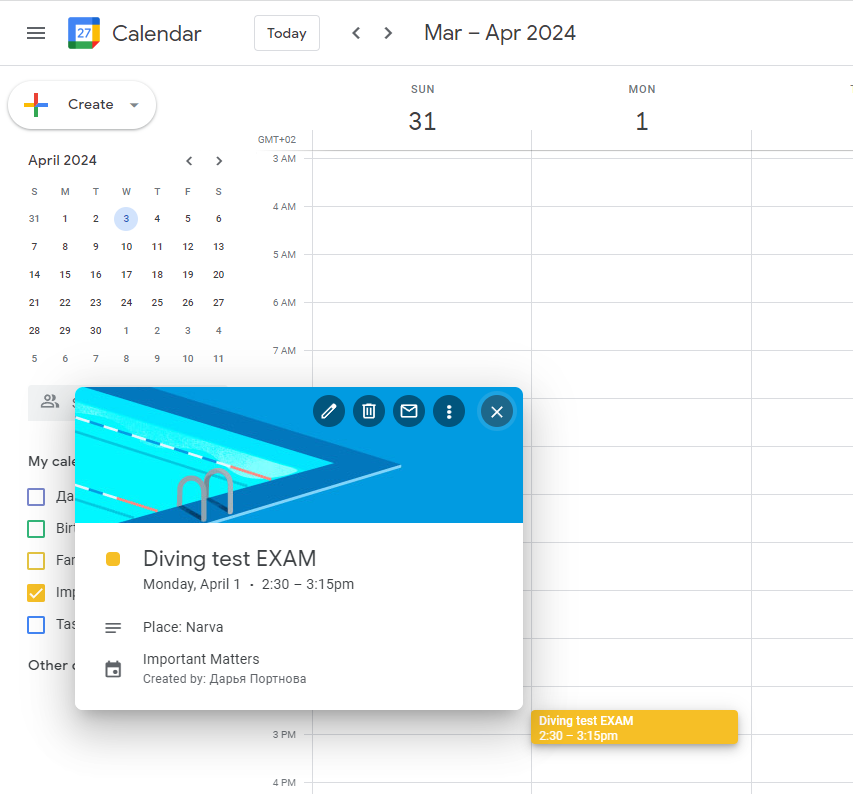
Here’s how the result looks on the phone:
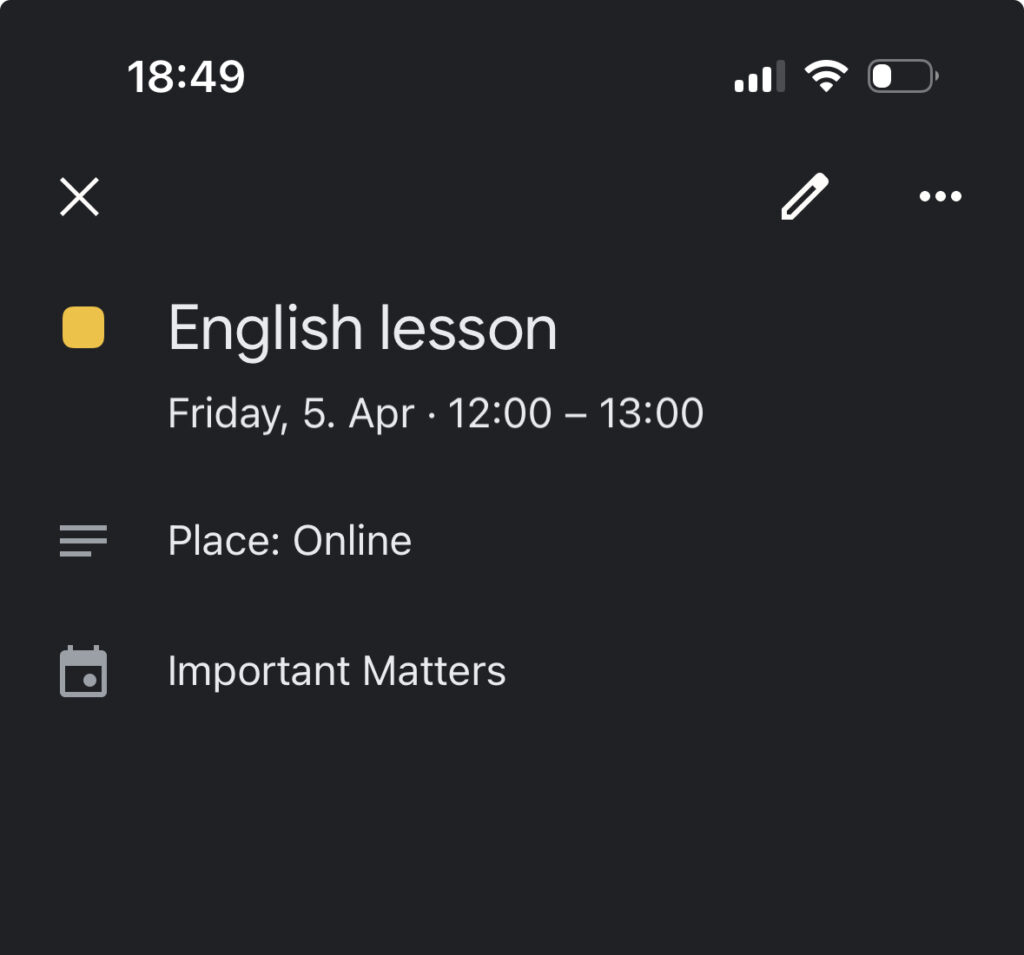
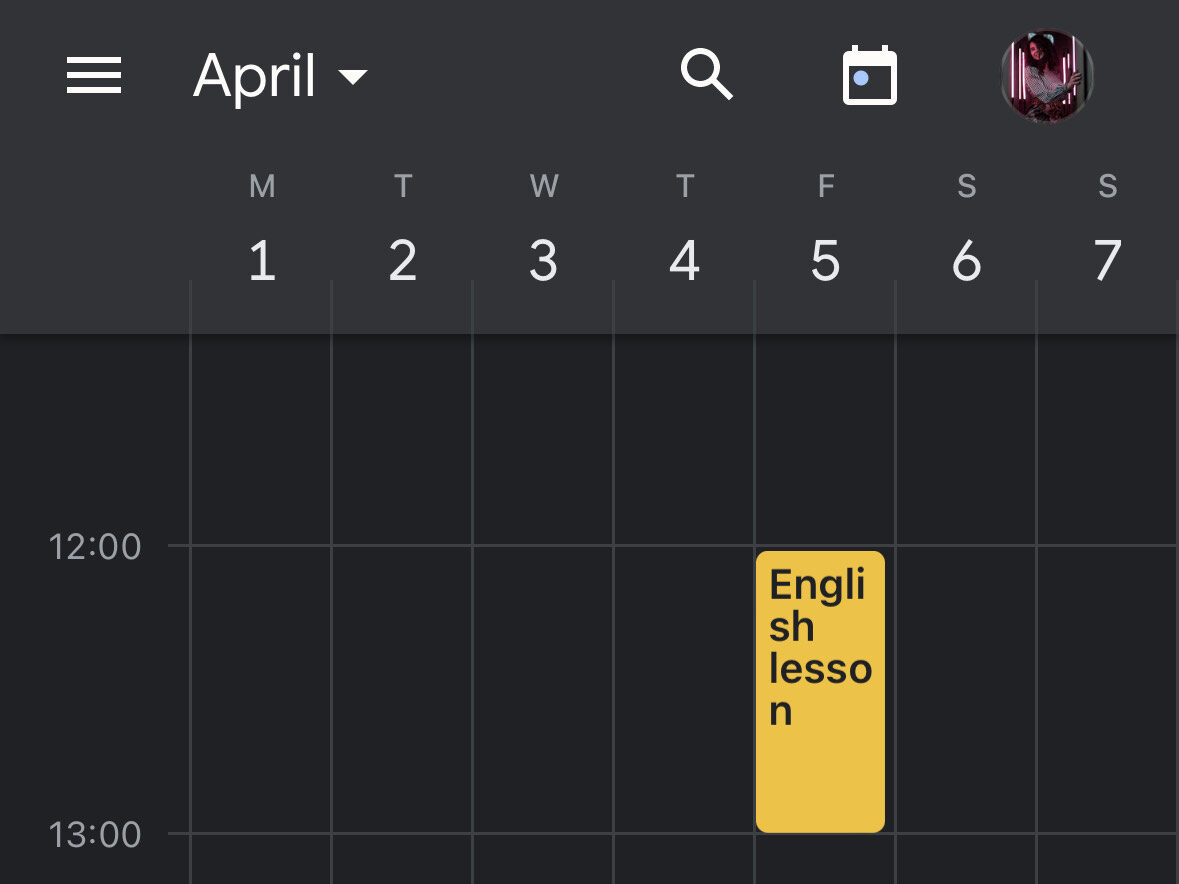