function onOpen() {
SpreadsheetApp.getUi()
.createMenu('Custom Menu')
.addItem('Show alert', 'showAlert_')
.addItem('Show prompt', 'showPrompt_')
.addToUi();
}
https://developers.google.com/apps-script/reference/base/ui#alerttitle-prompt-buttons
https://developers.google.com/apps-script/reference/base/ui#prompttitle-prompt-buttons
Original version:
function showAlert() {
var ui = SpreadsheetApp.getUi();
var result = ui.alert(
'Confirm action', // Window title
'Are you sure you want to proceed?', // Message
ui.ButtonSet.YES_NO // Buttons
);
if (result == ui.Button.YES) {
// User clicked "Yes".
ui.alert(''Confirmation received.');
} else { // User clicked "No" or X (closed the window)
ui.alert('Permission denied.');
}
}
function showPrompt() {
var ui = SpreadsheetApp.getUi();
var result = ui.prompt(
'Share something about yourself!', // Title
'Enter your name:', // Message
ui.ButtonSet.OK_CANCEL // Buttons
);
var button = result.getSelectedButton(); // The button that the user clicked
var text = result.getResponseText(); // The text entered by the user
if (button == ui.Button.OK) {
ui.alert(Your name: ' + text + '.');
} else if (button == ui.Button.CANCEL) {
ui.alert('You refused to enter your name.');
} else if (button == ui.Button.CLOSE) {
ui.alert('You closed the window.');
}
}
My version with changes or enhancements:
function showAlert_() {
var ui = SpreadsheetApp.getUi();
var result = ui.alert(
'Confirm action',
'Are you sure you want to proceed?',
ui.ButtonSet.YES_NO
);
if (result == ui.Button.YES) {
ui.alert('Confirmation received.');
} else {
ui.alert('Permission denied.');
}
}
function showPrompt_() {
var ui = SpreadsheetApp.getUi();
var result = ui.prompt(
'Share something about yourself!',
'Enter your name:',
ui.ButtonSet.OK_CANCEL
);
var result2 = ui.prompt(
'Enter your age:',
ui.ButtonSet.OK_CANCEL
);
var button = result.getSelectedButton();
var text = result.getResponseText();
var button2 = result2.getSelectedButton();
var text2 = result2.getResponseText();
if (button == ui.Button.OK & button2 == ui.Button.OK) {
ui.alert('Your name: ' + text + '. Your age: ' + text2);
} else if (button == ui.Button.CANCEL || button2 == ui.Button.CANCEL) {
ui.alert('You refused to enter your name or age.');
} else if (button == ui.Button.CLOSE & button2 == ui.Button.CLOSE) {
ui.alert('You closed both windows.');
}
}
function showAlert_:
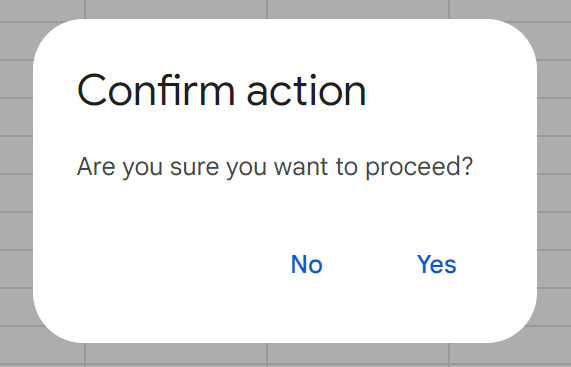
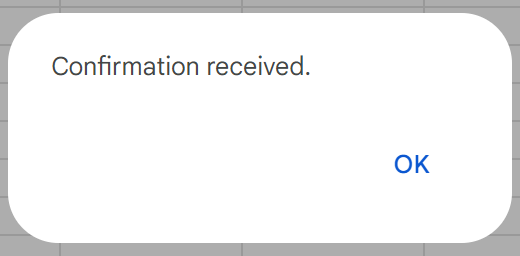
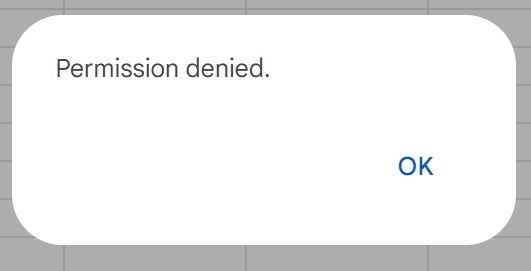
function showPrompt_:
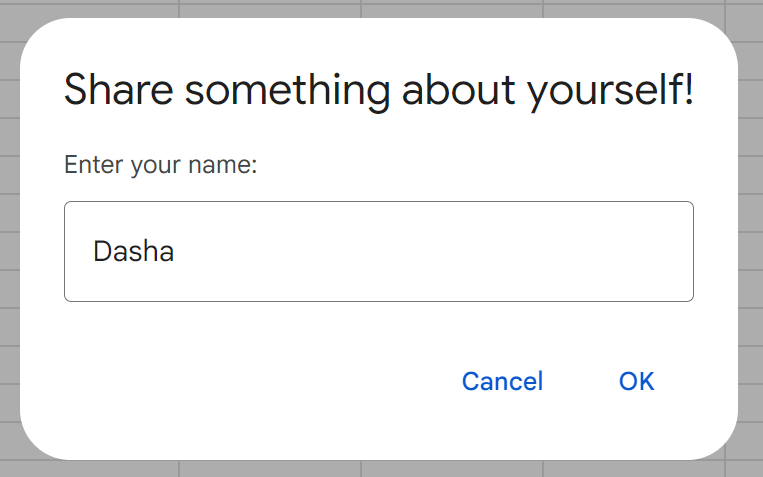

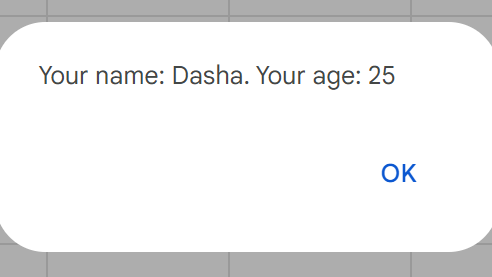
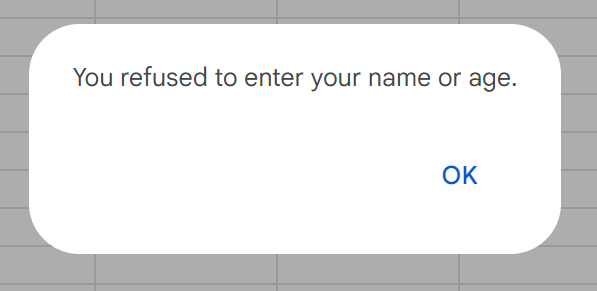