Class google.script.run (Client-side API):
https://developers.google.com/apps-script/guides/html/reference/run
‘Close Sidebar’ button is clicked:
https://developers.google.com/apps-script/guides/html/reference/host#close()
https://developers.google.com/apps-script/reference/base/ui#showsidebaruserinterface
Original version:
// Opening the sidebar
function showSidebar() {
// Including Bootstrap
var sidebarHTML = '<link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.3.1/css/bootstrap.min.css">';
// Including jQuery
sidebarHTML += '<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script>';
// Creating a form
sidebarHTML += '<form style="padding: 20px;text-align:center;">
<div class="form-group">
<label for="name">Name</label>
<input type="text" class="form-control" id="name" name="name" value="">
</div>
<div class="form-group">
<label for="abrakadabra">Some abrakadabra</label>
<textarea class="form-control" id="abrakadabra" name="abrakadabra" rows="3"></textarea>
</div>
<div class="form-group">
<label for="strNum">Row number to enter the data</label>
<input type="text" class="form-control" id="strNum" name="strNum" value="">
</div>
<button type="submit" class="btn btn-primary">Write Data to Table</button>
<br><br><br>
<button type="button" id="sidebarClose" class="btn btn-danger">Close Sidebar</button>
</form>';
// Adding scripts
// When the form is submitted, call the function writeStrInTable() and pass the entered data to it
// When the 'Close Sidebar' button is clicked, close it
sidebarHTML += "<script>
$(document).on('submit', 'form', function () {
google.script.run
.withSuccessHandler(function (resultMsg) {
alert(resultMsg);
})
.writeStrInTable(
{ name: $('#name').val(), abrakadabra: $('#abrakadabra').val(), strNum: $('#strNum').val() }
);
return false;
});
$('#sidebarClose').on('click', function() {
google.script.host.close();
});
</script>";
var htmlOutput = HtmlService
.createHtmlOutput(sidebarHTML)
.setTitle('My add-on');
SpreadsheetApp.getUi().showSidebar(htmlOutput);
}
function writeStrInTable(e) {
var name = e.name;
var abrakadabra = e.abrakadabra;
var strNum = parseInt(e.strNum);
// Getting the object of the active (currently open) table
var ss = SpreadsheetApp.getActiveSpreadsheet();
var sheet = ss.getActiveSheet();
// Writing the received data to the table
sheet.getRange("A" + strNum).setValue(name);
sheet.getRange("B" + strNum).setValue(abrakadabra);
return "Everything went smoothly!";
}
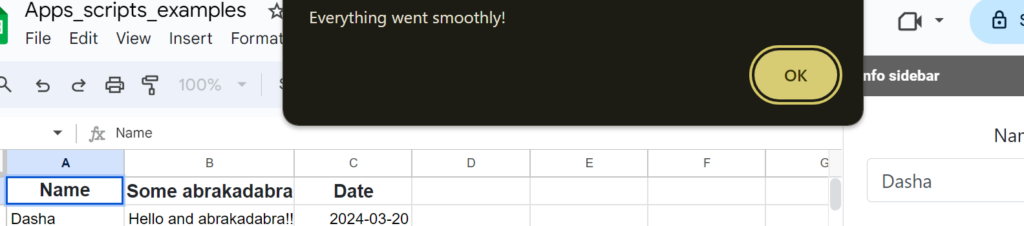
My version with changes or enhancements:
function showSidebar_() {
// bootstrap and jQuery and creating form
var sidebarHTML = '<link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.3.1/css/bootstrap.min.css">';
sidebarHTML += '<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script>';
sidebarHTML += '<form style="padding: 20px;text-align:center;">
<div class="form-group">
<label for="name">Name</label>
<input type="text" class="form-control" id="name" name="name" value="">
</div>
<div class="form-group">
<label for="abrakadabra">Some abrakadabra</label>
<textarea class="form-control" id="abrakadabra" name="abrakadabra" rows="3"></textarea>
</div>
<div class="form-group">
<label for="strNum">Row number to enter the data</label>
<input type="text" class="form-control" id="strNum" name="strNum" value="">
</div>
<div class="form-group">
<label for="date">Date</label>
<input type="date" class="form-control" id="date" name="date" value="">
</div>
<button type="submit" class="btn btn-primary">Write Data to Table</button>
<br><br><br>
<button type="button" id="sidebarClose" class="btn btn-danger">Close Sidebar</button>
</form>';
sidebarHTML += "<script> // + scripts
$(document).on('submit', 'form', function () {
google.script.run
.withSuccessHandler(function (resultMsg) {
alert(resultMsg);
})
.writeStrInTable(
{ name: $('#name').val(), abrakadabra: $('#abrakadabra').val(), date: $('#date').val(), strNum: $('#strNum').val() }
);
return false;
});
$('#sidebarClose').on('click', function() {
google.script.host.close();
});
</script>";
var htmlOutput = HtmlService
.createHtmlOutput(sidebarHTML)
.setTitle('Info sidebar');
SpreadsheetApp.getUi().showSidebar(htmlOutput);
}
function writeStrInTable(e) {
var name = e.name;
var abrakadabra = e.abrakadabra;
var date = e.date;
var strNum = parseInt(e.strNum);
var ss = SpreadsheetApp.getActiveSpreadsheet(); // get object
var sheet = ss.getActiveSheet(); // get active table
// Write Data to Table
sheet.getRange("A" + strNum).setValue(name);
sheet.getRange("B" + strNum).setValue(abrakadabra);
sheet.getRange("C" + strNum).setValue(date);
return "Everything went smoothly!";
}
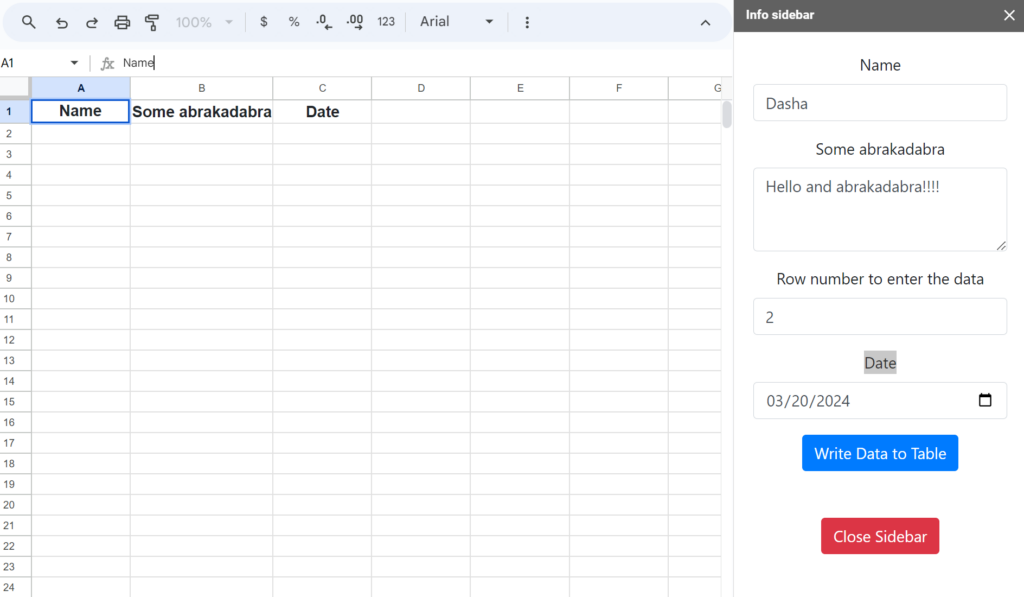
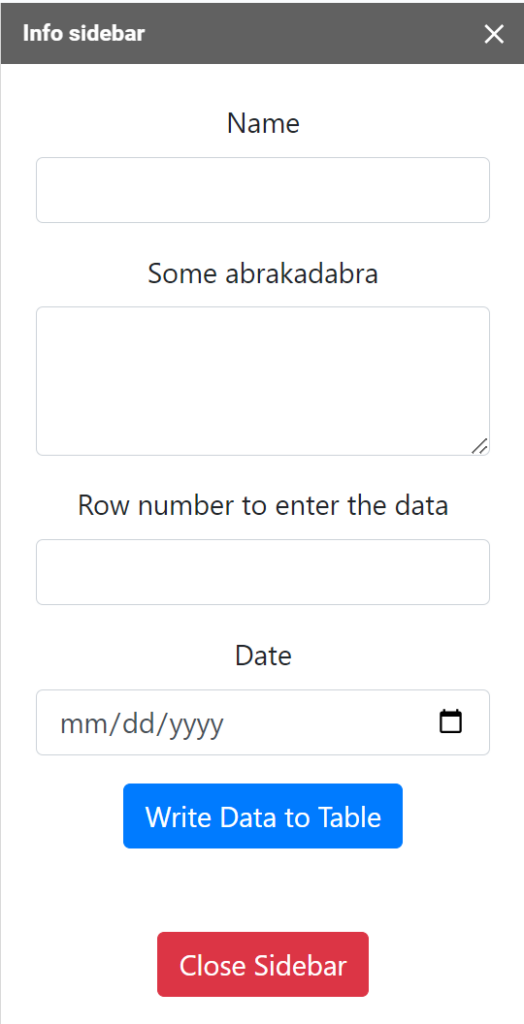