This program helps in opening and closing a garage door based on the measured distance and controls LEDs and a buzzer for feedback. It uses a servo motor, a buzzer, and a light sensor to manage the garage door and displays “Have a good trip” and the outside temperature on an LCD screen. When the door opens and closes, the buzzer makes a sound. There are two RGB lights: red means you cannot go (like a traffic light), and green means you can drive now. Additionally, a white light will turn on when it is dark.
#include <Servo.h>
#include <LiquidCrystal.h>
#define TRIG_PIN 7
#define ECHO_PIN 6
Servo myservo;
const int tempPin = A0;
const int lightPin = A1;
const int buzzerPin = 9;
const int servoPin = 8;
const int redPin = 13;
const int greenPin = 10;
const int whitePin = 1;
int distance;
int duration;
const float calibration = 0.1039;
LiquidCrystal lcd(12, 11, 2, 3, 4, 5);
void setup() {
pinMode(ECHO_PIN, INPUT);
pinMode(TRIG_PIN, OUTPUT);
pinMode(buzzerPin, OUTPUT);
myservo.attach(servoPin);
myservo.write(0);
pinMode(redPin, OUTPUT);
pinMode(greenPin, OUTPUT);
pinMode(whitePin, OUTPUT);
lcd.begin(16, 2);
delay(1000);
}
void loop() {
digitalWrite(TRIG_PIN, LOW);
delayMicroseconds(2);
digitalWrite(TRIG_PIN, HIGH);
delayMicroseconds(10);
digitalWrite(TRIG_PIN, LOW);
duration = pulseIn(ECHO_PIN, HIGH);
distance = duration / 58.2; // cm
float temperature = analogRead(tempPin) * calibration;
displayTemp(temperature);
delay(100);
//garage door will open if distance between 0 and 50 cm
if (distance > 0 && distance < 50) {
departure(temperature);
}
delay(100);
//if light level is low, show temperature on the LCD
int lightLevel = analogRead(lightPin);
if (lightLevel < 155) {
digitalWrite(whitePin, HIGH);
} else {
digitalWrite(whitePin, LOW);
}
}
void departure(float temp) {
makeSound();
delay(100);
lcd.clear();
lcd.setCursor(1, 0);
displayTemp(temp);
lcd.setCursor(0, 1);
lcd.print("Have a good trip");
lightOn("opening");
openGarageDoor();
lightOn("open");
delay(5000); // keep opened for 5 seconds
lightOn("closing");
makeSound();
delay(100);
closeGarageDoor();
delay(1000); // give some time before marking closed
lightOn("closed");
}
void displayTemp(float temp) {
lcd.clear();
lcd.setCursor(1, 0);
lcd.print("Temp: ");
lcd.print(temp);
lcd.print(" C");
}
void makeSound() {
for (int i = 0; i < 3; i++) {
tone(buzzerPin, 1000);
delay(1000);
noTone(buzzerPin);
delay(800);
}
}
void openGarageDoor() {
myservo.write(90);
}
void closeGarageDoor() {
myservo.write(0);
}
void lightOn(String state) {
if (state == "closed") {
digitalWrite(redPin, HIGH);
digitalWrite(greenPin, LOW);
} else if (state == "opening" || state == "closing") {
for (int i = 0; i < 3; i++) {
digitalWrite(redPin, HIGH);
digitalWrite(greenPin, LOW);
delay(10);
digitalWrite(redPin, LOW);
}
} else if (state == "open"){
digitalWrite(greenPin, HIGH);
digitalWrite(redPin, LOW);
delay(5000);
}
}
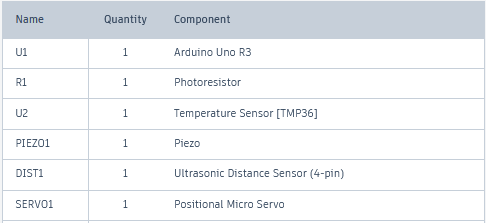
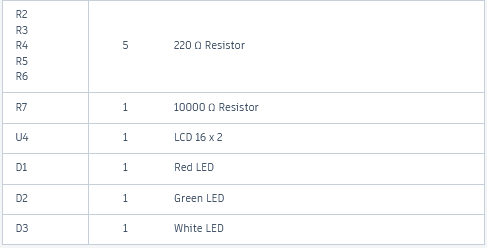

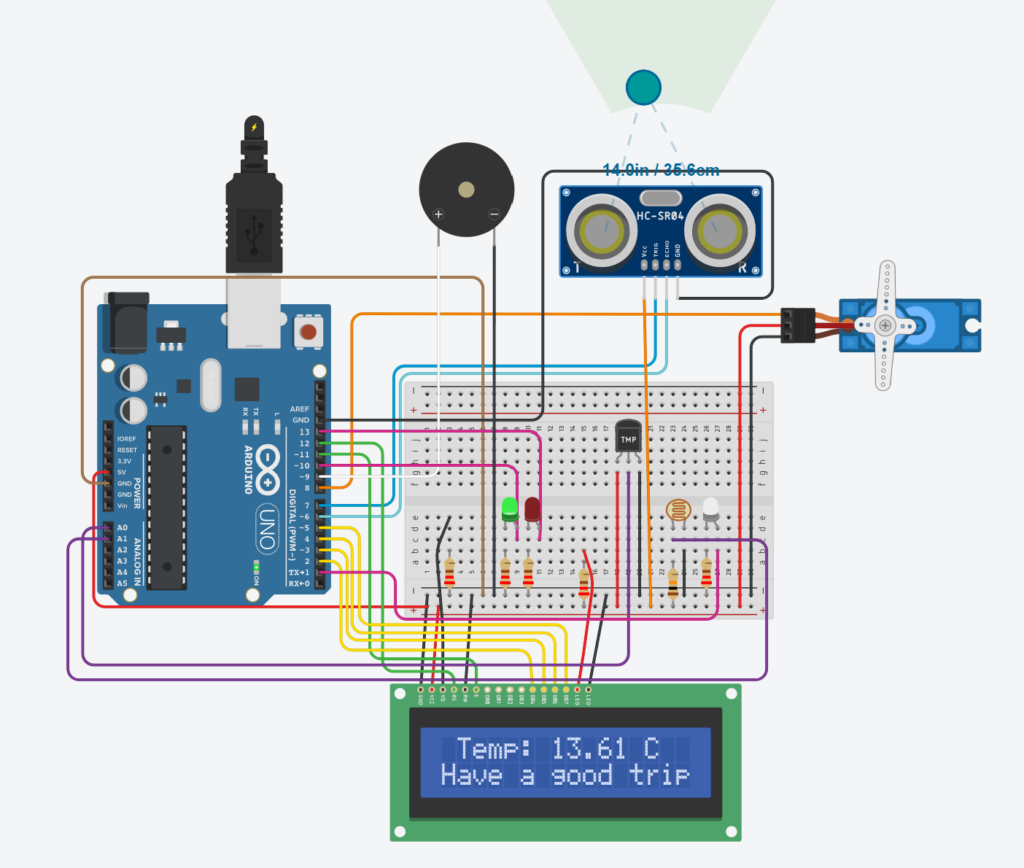
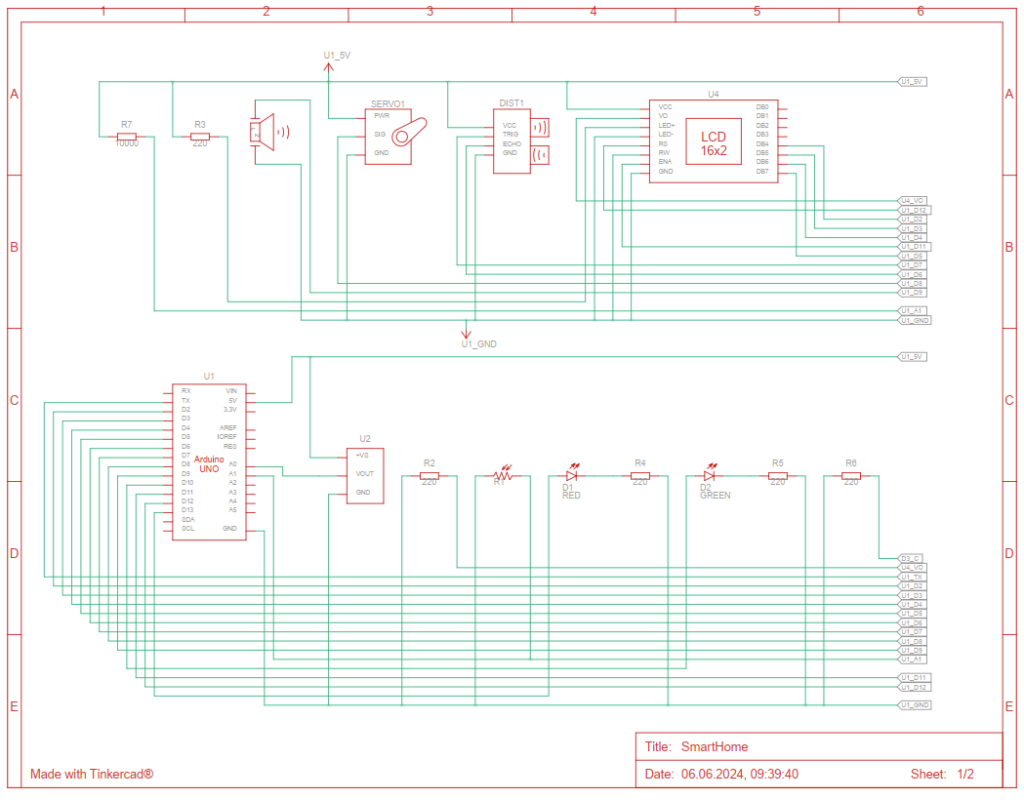
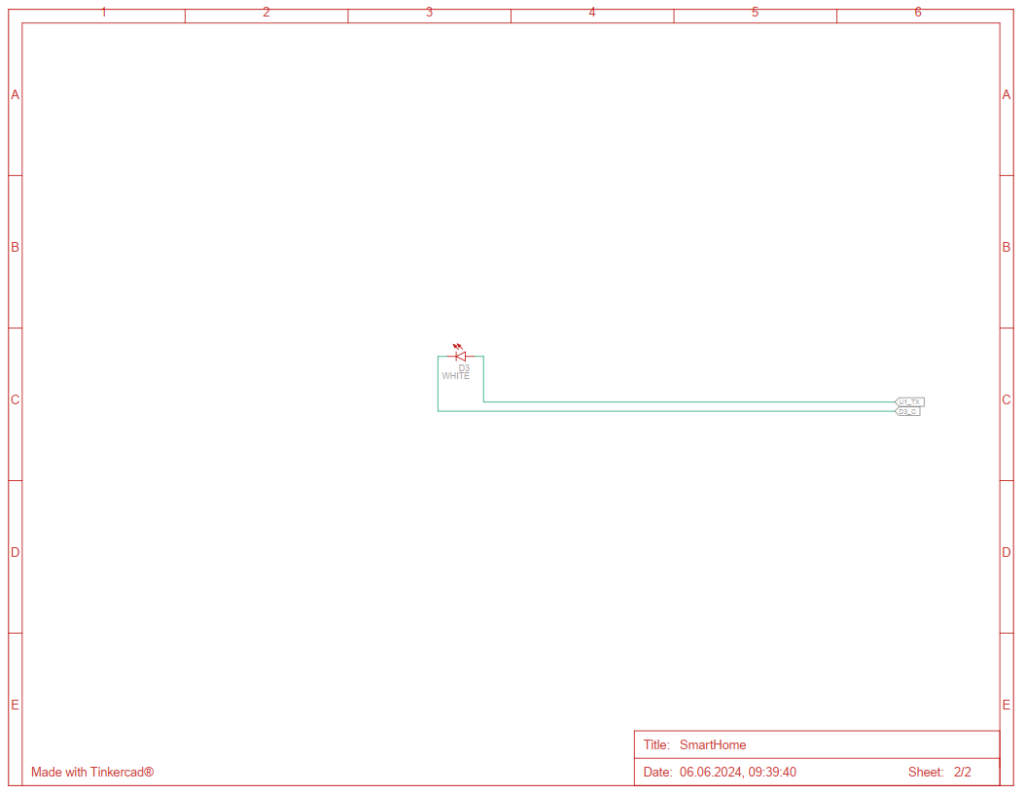
- Libraries for the servo motor and LCD screen
- It defines pins for the ultrasonic sensor (TRIG_PIN and ECHO_PIN), temperature sensor (tempPin), light sensor (lightPin), buzzer (buzzerPin), servo motor (servoPin), and LEDs (redPin, greenPin, whitePin)
- Setup Function:
- Sets pin modes
- Attaches the servo motor
- Initializes the LCD screen
- Loop Function:
- Measures the distance using the ultrasonic sensor
- Reads the temperature and light level
- Opens the garage door if the distance is between 0 and 50 cm
- Turns on the white LED if the light level is low
- Helper Functions:
departure
: manages the garage door opening and closing process, displays a message on the LCD, and controls the LEDsdisplayTemp
:displays the temperature on the LCDmakeSound()
: makes a beeping sound using the buzzeropenGarageDoor()
: opens the garage door by setting the servo to 90 degreescloseGarageDoor()
: closes the garage door by setting the servo to 0 degreeslightOn
: controls the red and green LEDs based on the state of the garage door (closed, opening, open, closing)